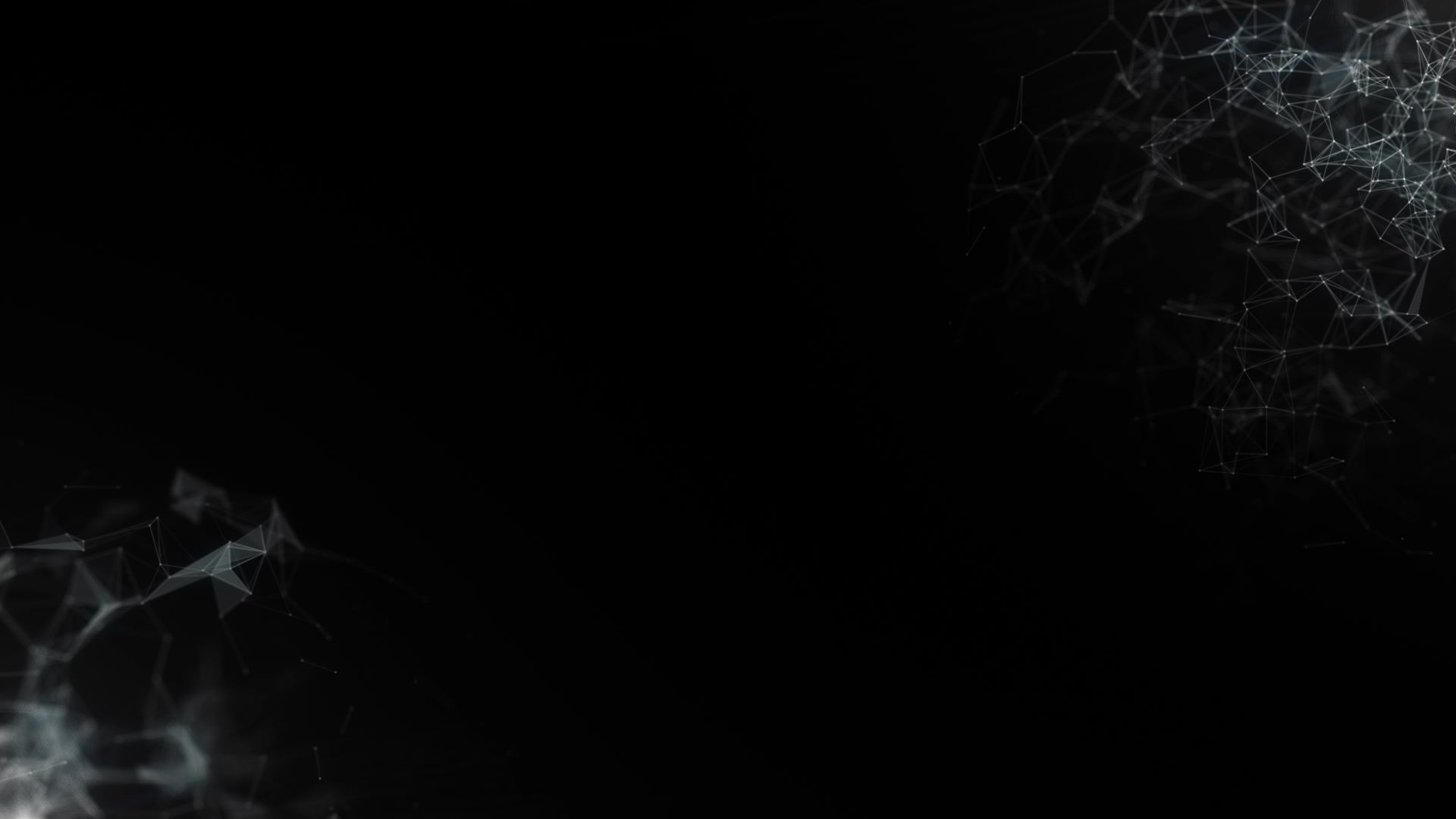
SP: Utility Function Library
This is the documentation hub for the Shady Practices: Utility Function Library for Unreal Engine 4.
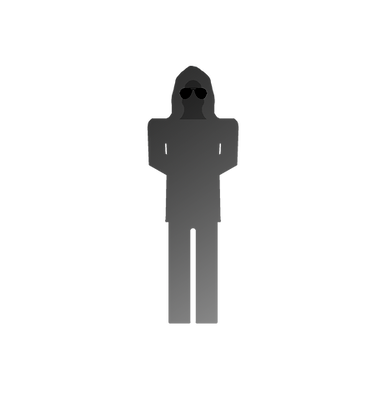
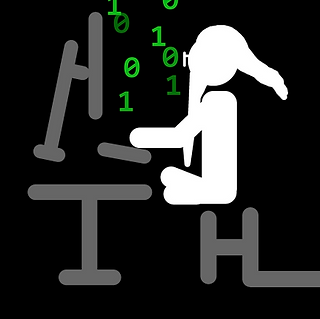
Below is a comprehensive list of all of the current functionality offered in the library:
- Check in Front
- Get Furthest Actor of Class
- Get Furthest Instance
- Get Closest Actor of Class
- Get Closest Instance
- Is in Front of Actor?
- Get Distance to Point
- Can See Actor?
- Get Angle Between Actors
- Is Right of Actor?
- Get Random Point On Circle
- Get Random Point on Sphere
- Random Color
- Random Color Opaque
- Random Linear Color
- Random Linear Color Opaque
- Add to Angle
DOCS
- Check in Front -
Checks whether or not an actor is in front of another.
Parameters:
- Source: Vector location of where to begin checking in front of
- Source Forward: The forward vector of the given source point
- Target: The target vector location that you want to determine if it is in front of the source or not
- Max Angle:
- Debug: Whether or not to show debug
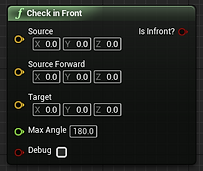
- Get Furthest Instance -
Returns the furthest away actor in the given array as well as the distance in cm from that actor to the origin point.
Parameters:
- Origin: Vector location of where you are checking distances from
- Use XY?: If checked, ignores the Z axis distance
- Squared: Check this if the distance returned does not matter and you want higher performance
- Debug: Whether or not to show debug
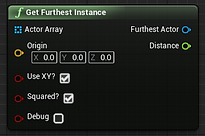
- Get Furthest Actor of Class -
- Get Closest Instance -
Returns the furthest away actor of a given class to a specified point.
Do not use this on tick as it can be very expensive.
Parameters:
- Origin: Vector location of where you are checking distances from
- Use XY?: If checked, ignores the Z axis distance
- Squared: Check this if the distance returned does not matter and you want higher performance
- Debug: Whether or not to show debug

Returns the closest actor in the given array as well as the distance in cm from that actor to the origin point.
Parameters:
- Origin: Vector location of where you are checking distances from
- Use XY?: If checked, ignores the Z axis distance
- Squared: Check this if the distance returned does not matter and you want higher performance
- Debug: Whether or not to show debug
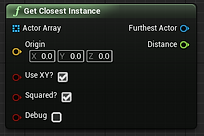
- Get Closest Actor of Class -
Returns the closest actor of a given class to a specified point.
Do not use this on tick as it can be very expensive.
Parameters:
- Actor Class: The specified class to find an instance of
- Origin: Vector location of where you are checking distances from
- Use XY?: If checked, ignores the Z axis distance
- Squared: Check this if the distance returned does not matter and you want higher performance
- Debug: Whether or not to show debug

- Is In Front of Actor -
Returns true if Other Actor is in front of Actor, and false if it is not.
Parameters:
- Actor: Vector location of where you are checking distances from
- Other Actor: The other actor that is either in front of or behind Actor
- In Front Angle: The angle in degrees that you want
- Use XY?: If checked, ignores the Z axis distance
- Debug: Whether or not to show debug
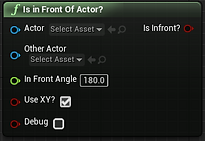
- Get Distance to Point -
Returns the distance between two vector locations in cm.
Parameters:
- Source: Vector location start
- Target: Vector location end
- Use XY?: If checked, ignores the Z axis distance
- Distance Squared?: Returns the distance between points squared if true (better for performance)
- Debug: Whether or not to show debug
- Debug Draw Duration: The duration in seconds that debug is drawn for

- Can See Actor -
Returns true if the Target actor is visible from Eye Location, false if it is not.
Pass an empty array into Actors to Ignore if you do not want to ignore any actors blocking visibility.
Parameters:
- Eye Location: Vector location of the "eyes"
- Target: The actor you want to see from Eye Location
- Actors to Ignore: An array of actors to ignore
- Debug: Whether or not to show debug
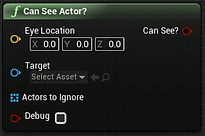
- Get Angle Between Actors -
Returns the angle between two actors in degrees or radians. Compares using their forward vectors.
Parameters:
- Actor: Actor A
- Other Actor: Actor B
- Degrees?: An array of actors to ignore
- Debug: Whether or not to show debug
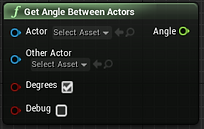
- Is Right of Actor? -
Returns true if Other Actor is on the right side of Actor. Uses Actor's forward vector.
Parameters:
- Actor: Actor A
- Other Actor: Actor B

- Get Random Point on Circle -
Returns a random location as a vector that lies on a specified circle.
Parameters:
- Origin: The location of the center of the circle
- Radius: The half-width of the circle in cm
- Y Axis: The Y-Axis of the circle in vector form
- Z Axis: The Z-Axis of the circle in vector form
- Debug: Whether or not to show debug
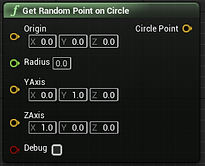
- Get Random Point on Sphere -
Returns a random location as a vector that lies on the surface of a specified sphere.
Parameters:
- Origin: The location of the center of the sphere
- Radius: The half-width of the sphere in cm
- Debug: Whether or not to show debug

- Random Color -

Returns a random RGB color with a random Alpha value between 50 and 255 to ensure visibility.
Parameters:
Returns a random RGB color with an Alpha value of 255.
Parameters:
- Random Color Opaque -

- Random Linear Color -
Returns a random RGB linear color with a random Alpha value between 1 and 0.
Parameters:
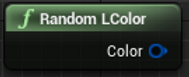
- Random Linear Color Opaque -
Returns a random RGB linear color with an Alpha value of 1.
Parameters:
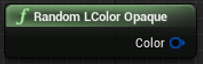
- Add to Angle -
Adds two angles together wrapping around between 180 and -180 degrees.
Parameters:
- Angle: The initial angle to add to
- New Degrees: The amount you want to add to the angle in degrees
- Degrees: If checked will return the result in degrees, if unchecked the result will be in radians

Questions or Concerns? Email me us at shadypracticestudios@gmail.com