// You are given n numbers as well as n probabilities that sum up to 1.
// Write a function to generate one of the numbers with its corresponding probability.
// For example, given the numbers[1, 2, 3, 4] and probabilities[0.1, 0.5, 0.2, 0.2],
// your function should return 1 10 % of the time, 2 50 % of the time, and 3 and 4 20 % of the time.
// You can generate random numbers between 0 and 1 uniformly.
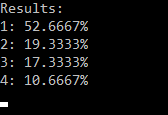
#include <iostream>
#include <string>
#include <vector>
#include <stdlib.h> /* srand, rand */
#include <time.h> /* time */
#include <algorithm> /* sort */
using namespace std;
struct Greater
{
template<class T>
bool operator()(T const &a, T const &b) const { return a > b; }
};
// returns the index of the randomly chosennumber
int randomNumber(vector<int> nums, vector<float> probs)
{
// first we want to sort probabilities from highest to lowest
sort(probs.begin(), probs.end(), Greater());
//srand(time(NULL));
float r = rand() % 100 + 1;
r = (float) r / 100.0f;
float total = 0.0f;
int i = 0;
while (i < nums.size())
{
total += probs[i];
if (r <= total)
return i; // return the index, not the number itself
++i;
}
return -1; // error
}
int main()
{
vector<float> probabilities = { 0.1f, 0.5f, 0.2f, 0.2f };
vector<int> nums = { 1, 2, 3, 4 };
vector<int> results = vector<int>(nums.size(), 0);
int tests = 300;
int result = 0;
for (int i = 0; i < tests; ++i)
{
result = randomNumber(nums, probabilities);
++results[result];
}
cout << "Results: " << endl;
for (int i = 0; i < results.size(); ++i)
{
cout << nums[i] << ": " << ((float)results[i] / (float)tests) * 100.0f << "%" << endl;
}
return 0;
}
Comments